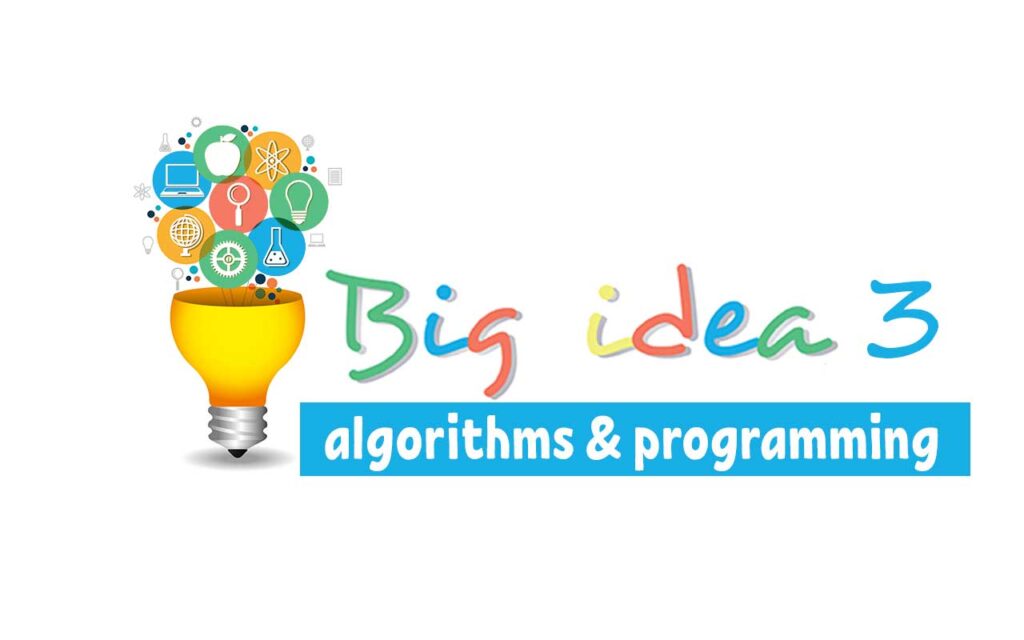
Exploring AP CSP Big Idea 3: Understanding Algorithms and Programming
programming concepts
programming abstraction
AP CSP Exam Questions: Unit 3 Study Guides
These resources are for students taking ap computer science principles to help for study and exam preparation.
Remember that there are 70 multiple-choice questions and big ideas 3 takes up about a third of these questions. Therefore, although there are 18 programming areas, there will only be one or two questions from each area.
Below, there is an extensive range of question types that could be used in the exam. But, there will only be one or two questions from each area.
Programming Fundamentals
These are types of questions from the simple areas of programming in the ap csp exam.
3.1 Variables and Assignments
- A variable is a storage place for data.
- Use ← to assign values.
x ← 5
name ← "Alice"
- Variables can be updated
- x ← x + 2
- x is now 7 (it was 5)
Type of questions:
- Find the constant variable.
- What is the output of these variable values (e.g., x, y, z)?
- Choose a good name for a variable or a list.
- Which code correctly swaps x and y?
- What type of data should be stored?
- What are the correct data types?
- Use MOD (find the remainder).
- Choose the correct code for a given situation.
- Find 20% profit of x using y and z.
3.3 Mathematical Expressions
- Using basic arithmetic operators
- + – * /
- total ← 10 + 5
- Using MOD
- x← 10 MOD 3
- x is 1 (the remainder)
Type of questions:
- Explain this code (e.g., find the circumference).
- Rewrite this expression using PEMDAS.
- Use MOD (find the remainder).
- Find z, given x and y.
- Convert time between units.
- What is the output (nothing is displayed)
- Solve this complex expression: result = a + b / c * b MOD c.
- Calculate the total time.
3.4 Strings
- A string is text enclosed in quotes.
- Concatenation
- greeting ← “Hello” + ” World”
- DISPLAY(greeting)
- the output is “Hello World”
- Finding length
- length ← LEN(“apple”)
- The length is 5
- apple is 5 characters long
Type of questions:
- Using string functions
- substring
- length
- append
- Concatenation (using + or join)
- Using strings with advanced functions
- subword,
- index,
- random,
- nested if statements,
- procedures,
AP Computer Science Principles Exam Preparation
Everything you need to prepare for the ap csp exam, including dates, practice questions, previous results and a score calculator chart. Good luck!
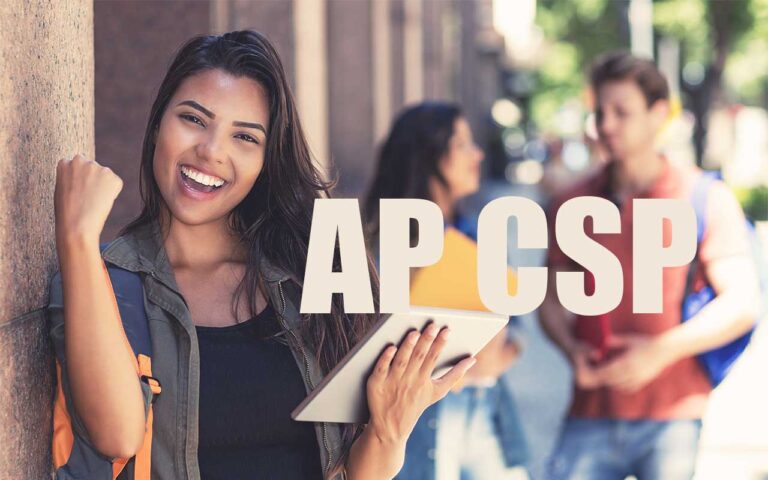
Programming Conditionals and Logic
These are types of questions from programming related to conditionals in Boolean values if statements and variants of the if statements that use else and nested if statements.
Logic includes the use of logic operators if True or False statements (i.e. AND, OR and NOT), and the robot movement problems also seen in the a csp exam.
3.5 Boolean Expressions
- Boolean values
- TRUE or FALSE
- Comparison operators
- = (equal)
- ≠ (not equal)
- > (greater)
- < (less)
- x ← 5
- DISPLAY(x > 3)
- TRUE
- The Boolean true applies as x is greater than 3
- Logical operators
- AND, OR, NOT
Type of questions:
- Find the line of code with a Boolean expression.
- Which code checks if x is between 10 and 20?
- Boolean with logical operators (e.g., AND, OR).
- Is this Boolean value True or False?
- Identify nested Boolean expressions.
- Find which expressions give the same result:
- Without logical operators
- With logical operators
3.6 Conditionals
Type of questions:
- Choose the correct statement.
- Choose the correct condition (e.g., update high score).
- Selection
- Using a Boolean
- What is the output?
- False condition
- If-else
- Code change
- Output (multiple if-else)
3.7 Nested Conditionals
Type of questions:
- Nested if statements
- Nested if
- Nested if-else
- Change if-else code
- Identify the error – an impossible condition
- Check if a condition is correct (e.g., over 18 AND …)
- Is this nested if-else correct?
- What is the output for a given input?
- Describe the result
- Do these nested if-else statements give the same output?
- Multiple nested if-else statements
Other Programming Logic
In the exam, there are two types of questions that are not specifically covered in the 18 areas:
- Which set of instructions moves the robot from the start square to the end position?
- True or False expressions that use multiple parts, including True, False, AND, NOT, and OR
Programming Concepts
These are several areas of programming in the ap csp multiple choice questions including these areas we have grouped here.
3.8 Iteration
Type of questions:
- Repeat loop – what is the output
- Repeat loop – if condition is value what is the output
- Repeat loop with a condition using MOD what is the output
- Repeat until loop – what is the output
- Descibe the problem (assignment (4) /condition (=0) unmatched)
- Which options are iteration
- Select the correct condition in this repeat loop
- How many iterations (loops) does this repeat until loop do
- Repeat loop with a condition using MOD to describe the output
- What changes give the repeat loop the same output as the original code
- What is the output of this code that has an inner loop inside an outer loop
- Which option keeps the same output of this procedure that contains a loop
- A procedure with a repeat loop describes the output
3.14 Libraries
Type of questions:
- What term is used for code stored for re-use
- Why are libraries useful
- Why do APIs and libraries need documentation
- Math is a library, which procedure is from this library
- Select the best API to work with the code
- Select the best library to improve the code
3.15 Random Values
Type of questions:
- Add the missing condition to the code that used RANDOM
- Add the missing code (use RANDOM correctly)
- Which numbers could be returned with RANDOM (5,10)
- Select the best procedure (uses RANDOM)
- Which option uses RANDOM that has two output possibilities
- Select the option that is NOT the output
- Which amendment corrects the code with RANDOM
- Input numbers using RANDOM in a ‘contains x’ procedure, which option is the correct code
3.16 Simulations
Type of questions:
- Which is the best option for input for this simulation experiment
- Which is the least good option for input for this simulation experiment
- Which options would be the best approach for this simulation experiment
- Weather forecast, why use this simulation
- Weather forecast, what is true of this simulation
- Weather forecast, what is the limitation of this simulation
- Which are the advantages of this simulation
- Which statement can be made about a simulation experiment
- Simulate the results of choosing a playing card, what is the output
- Simulate the results of rolling two dice 25 times, what are the likely outputs
- Simulate the results of student tests, amend the code mistake
Programming Data Abstraction
Data abstraction is a simple programming concept but often confusing to some students. In the ap csp course the primary area of abstraction is the use of lists and procedures.
3.2 Data Abstraction
- Abstraction means hidden detail to make something simpler
- Lists use abstraction to hide the items
- Procedures use abstraction to hide the inner code
- Lists store multiple values
- students ← [“Alice”, “Bob”, “Charlie”]
- Accessing an item (lists start at 1!):
- DISPLAY(students[1])
- The output is “Alice”
Type of questions:
- Which of the following is an example of abstraction?
- Name the computer principle.
- Which is the highest level of abstraction?
- Which two options are desired properties of procedural abstraction?
- Which is NOT a process of procedural abstraction?
- Why is this an example of abstraction?
- Which parts of the description are an abstraction?
- What is the best description of how we use abstraction in computing?
3.10 Lists
Type of questions:
- What is data abstraction (ADT)?
- Loop
- Use MOD (find the remainder)
- Choose or change a list value within a loop
- Display a list but skip one value
- Count even numbers in a list
- Index
- Choose or change a list value with an even-numbered index
- Choose or change a list value based on x
- Algorithm
- Find the lowest or highest element
- Find the lowest or highest element if 0 or more
- Iterate and change values using remove and append
Questions often include a procedure to make them harder
3.12 Calling Procedures
Type of questions:
These questions are primarily about using correct parameters
- Which option is not either an input or output of this procedure
- What happens when this procedure is called
- Which is the correct code for this output
- Factorial procedure, how can testing find it is not correct on all numbers
- Which option is an incorrect option about the procedure (based on the parameters)
- What will be displayed, given these input values
- What is the final robot position for this robot director procedure and input
- Which procedure has an error (based on the parameters)
- What happens with this procedure
- Convert temperature procedure, which is correct
- Select the correct code for this drawing procedure
- Given the goal, select the correct procedure with the correct parameters
- What are the input values for this robot director procedure
- Select the correct output
- Which is the correct input for this output
3.13 Developing Procedures
Type of questions:
There is a wide range of questions to developing procedures that are based on the knowledge of programming and coding, using pseudocode.
Algorithms
Also included in programming are algorithms. These are used occasionally as part of the abstraction questions but also by themselves in the ap csp exam.
3.9 Developing Algorithms
Type of questions:
- What does this line of code do (e.g., is it iteration or selection)?
- Which line of code is iteration or selection?
- Compare algorithms.
- Find errors, check input/output, or change lines of code in an algorithm.
- Solve problems such as:
- Find the highest number
- Counting, average
- Ordering (including descending order)
- Drawing shapes
- Random numbers
- Factorial
Questions may be written in:
- Normal text
- Code (pseudocode)
- A diagram (pseudocode or flowchart)
3.11 Binary Search
Type of questions:
- Describe binary search.
- Which is the best search approach?
- Describe the difference between binary and linear search.
- Compare different search approaches.
- Sorted vs. unsorted lists.
- Which is the best option for binary search?
3.17 Algorithmic Efficiency
Type of questions:
- Heuristics questions (e.g., what is the purpose, is each option true or not).
- Optimization questions, including:
- Define optimization.
- Consider optimization approaches.
- What are problems with this algorithm?
- Run time questions (e.g., is this true? Is this unreasonable?).
- Algorithm optimization for:
- Data compression
- Password cracking
- Traveling salesman problem
- Change code to improve efficiency (e.g., reduce loops, use nested if-else statements).
3.18 Undecidable Problems
Type of questions:
- Is this algorithm decidable or undecidable
- What type of problem is an algorithm
- if it only works on some data,
- tic-tac-toe,
- yes or no solution,
- Dijkstra’s algorithm,
- divides numbers to identify integers with a zero remainder
- Define an undecidable problem
- Choose the input that carries a concern of being an undecidable problem
Types of problems include: design, decision NP-ordinary, NP-complete, NP-hard (or not), and of course, decidable or undecidable.
Big Idea 3 FAQ
What are the three types of algorithms?
In AP CSP Unit 3 – Algorithms and Programming, three common types of algorithms include:
- Sequential algorithms – These execute steps in order, one after another.
- Conditional algorithms – These execute different blocks of code based on whether certain conditions are true or false.
- Iterative algorithms – These involve repetition, often running a specified number of times or until a desired element is found.
Is AP Computer Science Principles (AP CSP) hard to get a 5 on?
Scoring a 5 on the AP Exam requires strong problem-solving skills and a deep understanding of programming and algorithms. However, with practice, such as using flashcards, class notes, and step-by-step problem breakdowns, students can study with Quizlet and memorize key concepts effectively.
What are the big ideas in AP CSP?
The big ideas of AP CSP include:
- Computer systems and how they process data
- Algorithms and programming, focusing on the efficiency of algorithms, their impact on performance, and their applications to large datasets
- Data and analysis, including techniques like random number generation and search algorithms
- The Internet and cybersecurity, including how data is transmitted and protected
- The societal impact of computing, including ethical considerations
For more details on the big ideas visit our dedicated page, found here – AP CSP Big Ideas
Useful Information
- An algorithm can be written in many ways, but its efficiency depends on the size of the input and the resources needed to run it in terms of how long it will take and how much memory will be needed.
- Efficiency of algorithms deals with how quickly a solution is found and how much memory is used, especially when dealing with large datasets.
- Binary search is an efficient algorithm used for sorted lists, repeatedly dividing the search range until the desired element is found.
- Sequential search works best for small, unsorted lists but takes longer for larger datasets.
- Booleans are values that return true or false, often used to compare two values in conditions.
- Algorithms break down complex problems using strategies like divide and conquer, allowing for approximate solutions when exact solutions take too long.
- Random values can be used in simulations, while conditional statements control which code is executed when a condition is true.
- Flashcards containing terms like “element in the list,” “step-by-step processes,” and “return a boolean value” can help reinforce key programming concepts.
By mastering these concepts and practicing AP CSP Unit 3 – Algorithms and Programming, students can improve their understanding and performance on the AP Exam.